Chatty
Explore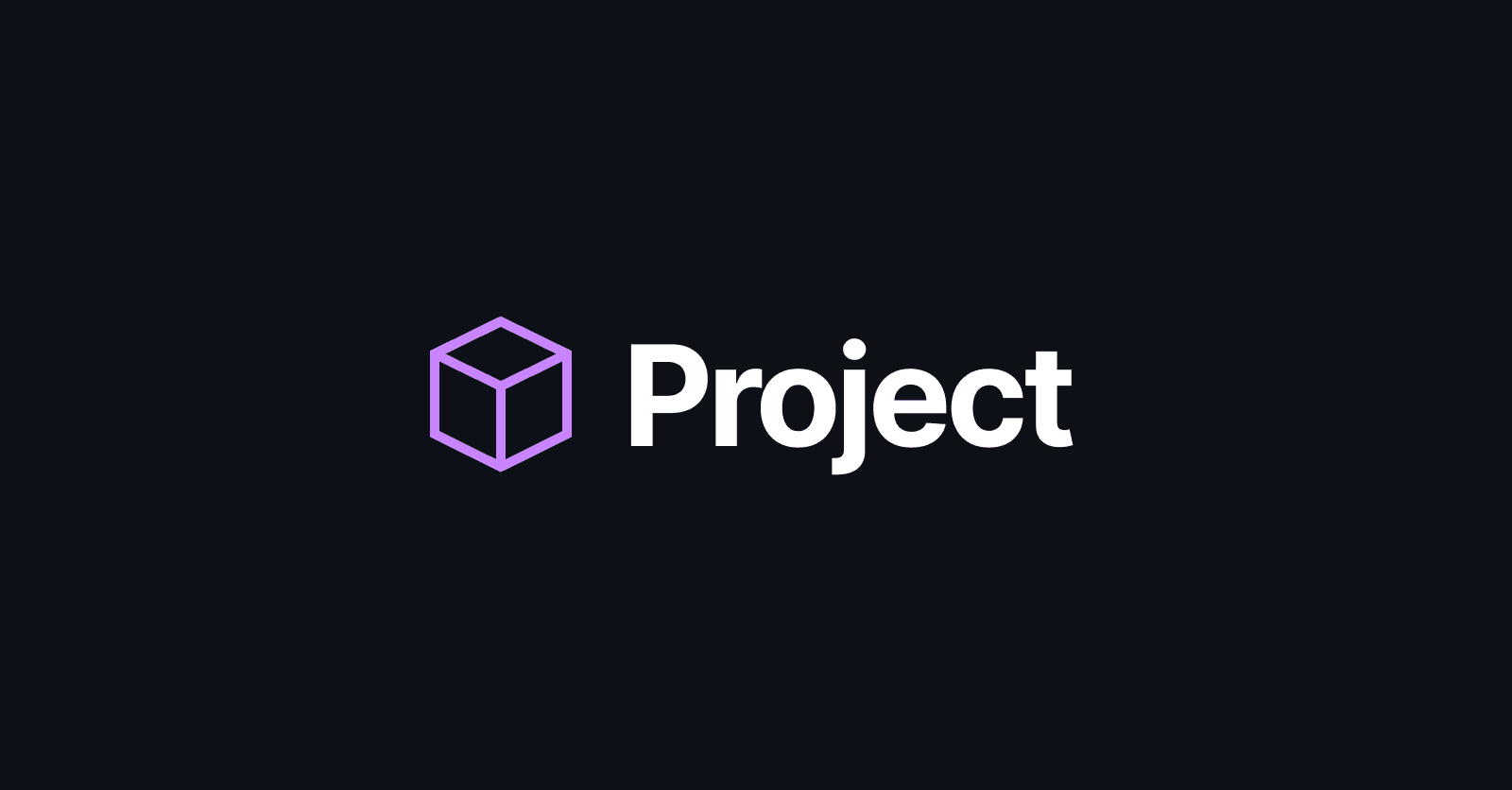
Project goal
This mobile app was developed for a university project during a cybersecurity course. The goal was to develop a chat application with encrypted messages by default like Signal or WhatsApp (fun fact: WhatsApp encryption is based on this library made by Signal).
The tech stack
After watching several courses on Youtube and articles on the internet I decided that using React Native could have been a great fit for the huge resources available. At the backend I decided to go with the solid Firebase services like Firestore and Storage for images, and to bring the functionalities of Firebase in our app we can use the rnfirebase library.
For all encryption related stuff we use a ported javascript library called tweetnacl. We could also use libsignal from Signal folks, which is more secure and sophisticated, but I decided to go with something simple and maybe explore that idea in the future.
Encryption
After a new user is created we store a randomly generated private key and public key with the SecureStorage library. This means that the user generated messages will be encrypted with that private key and if we try to use that account on another device all the messages will be lost because the key resides only on the device which has created it.
Sending the message
To send a message to all chat room users (single chat or groups) we first need to retrieve each user public key from the firestore DB. Then we can use tweetnacl to create a shared key between the sender and receiver. And finally we encrypt the message using the encrypt
utility function.
To encrypt the message we use the following code:
const encryptMessage = async publicKey => {
try {
const privateKey = await getMySecretKey(authUser.uid);
if (!privateKey) return;
// let's create the shared key between auth user and receiver
const sharedKey = await box.before(
stringToUint8Array(publicKey),
privateKey,
);
// encrypt message
const encryptedMessage = encrypt(sharedKey, {message});
return encryptedMessage;
} catch (e) {
console.log(e);
Alert.alert('A problem occurred while trying to encrypt message');
}
};
Receiving a message
The Message component is used to fetch an encrypted message from Firestore and then to decrypt its content. The following method is used:
const decryptMessage = async () => {
const myPrivateKey = await getMySecretKey(authUser.uid);
if (!myPrivateKey) return;
let sharedKey;
if (isMe) {
sharedKey = box.before(
stringToUint8Array(receiver.publicKey),
myPrivateKey,
);
} else {
sharedKey = box.before(
stringToUint8Array(sender.publicKey),
myPrivateKey,
);
}
const decrypted = decrypt(sharedKey, message.messageText);
setDecryptedContent(decrypted.message);
};
After retreiving the authenticated user private key, the shared key needs to be recalculated. This shared key differs whether the auth user was the sender or receiver. Finally we can use the decrypt function to get the plain text content.